这个着色器(最后的代码)使用 raymarching 来渲染程序几何:
但是,在图像(上图)中,背景中的立方体应该部分遮挡粉红色实体;不是因为这个:
struct fragmentOutput {
float4 color : SV_Target;
float zvalue : SV_Depth;
};
fragmentOutput frag(fragmentInput i) {
fragmentOutput o;
...
o.zvalue = IF(output[1] > 0, 0, 1);
}
但是,我终生无法弄清楚如何在此处正确生成深度值,以正确地允许光线行进的实体遮蔽/不遮蔽场景中的其他几何体。
我知道这是可能的,因为这里有一个工作示例:https://github.com/i-saint/RaymarchingOnUnity5 (相关的日语博客 http://i-saint.hatenablog.com/)
然而,它是日文的,而且基本上没有记录,而且非常复杂。
我正在寻找同一事物的极其简化的版本,以此为基础。
在着色器中,我目前正在使用片段程序行:
float2 output = march_raycast(i.worldpos, i.viewdir, _far, _step);
将需要相机(此着色器附加到它的相机)上的输入点 p 映射到输出 float2(密度,距离),其中距离是从四边形到程序表面上“点”的距离。
问题是,如何以任何有用的方式将其映射到深度缓冲区中?
完整的着色器在这里,要使用它,创建一个新场景,球体位于 0,0,0 处,大小至少为 50,并将着色器分配给它:
Shader "Shaders/Raymarching/BasicMarch" {
Properties {
_sun ("Sun", Vector) = (0, 0, 0, 0)
_far ("Far Depth Value", Float) = 20
_edgeFuzz ("Edge fuzziness", Range(1, 20)) = 1.0
_lightStep ("Light step", Range(0.1, 5)) = 1.0
_step ("Raycast step", Range(0.1, 5)) = 1.0
_dark ("Dark value", Color) = (0, 0, 0, 0)
_light ("Light Value", Color) = (1, 1, 1, 1)
[Toggle] _debugDepth ("Display depth field", Float) = 0
[Toggle] _debugLight ("Display light field", Float) = 0
}
SubShader {
Tags {"Queue"="Transparent" "IgnoreProjector"="True" "RenderType"="Transparent"}
Blend SrcAlpha OneMinusSrcAlpha
Pass {
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
#pragma target 3.0
#include "UnityCG.cginc"
#include "UnityLightingCommon.cginc" // for _LightColor0
#define IF(a, b, c) lerp(b, c, step((fixed) (a), 0));
uniform float _far;
uniform float _lightStep;
uniform float3 _sun;
uniform float4 _light;
uniform float4 _dark;
uniform float _debugDepth;
uniform float _debugLight;
uniform float _edgeFuzz;
uniform float _step;
/**
* Sphere at origin c, size s
* @param center_ The center of the sphere
* @param radius_ The radius of the sphere
* @param point_ The point to check
*/
float geom_soft_sphere(float3 center_, float radius_, float3 point_) {
float rtn = distance(center_, point_);
return IF(rtn < radius_, (radius_ - rtn) / radius_ / _edgeFuzz, 0);
}
/**
* A rectoid centered at center_
* @param center_ The center of the cube
* @param halfsize_ The halfsize of the cube in each direction
*/
float geom_rectoid(float3 center_, float3 halfsize_, float3 point_) {
float rtn = IF((point_[0] < (center_[0] - halfsize_[0])) || (point_[0] > (center_[0] + halfsize_[0])), 0, 1);
rtn = rtn * IF((point_[1] < (center_[1] - halfsize_[1])) || (point_[1] > (center_[1] + halfsize_[1])), 0, 1);
rtn = rtn * IF((point_[2] < (center_[2] - halfsize_[2])) || (point_[2] > (center_[2] + halfsize_[2])), 0, 1);
rtn = rtn * distance(point_, center_);
float radius = length(halfsize_);
return IF(rtn > 0, (radius - rtn) / radius / _edgeFuzz, 0);
}
/**
* Calculate procedural geometry.
* Return (0, 0, 0) for empty space.
* @param point_ A float3; return the density of the solid at p.
* @return The density of the procedural geometry of p.
*/
float march_geometry(float3 point_) {
return
geom_rectoid(float3(0, 0, 0), float3(7, 7, 7), point_) +
geom_soft_sphere(float3(10, 0, 0), 7, point_) +
geom_soft_sphere(float3(-10, 0, 0), 7, point_) +
geom_soft_sphere(float3(0, 0, 10), 7, point_) +
geom_soft_sphere(float3(0, 0, -10), 7, point_);
}
/** Return a randomish value to sample step with */
float rand(float3 seed) {
return frac(sin(dot(seed.xyz ,float3(12.9898,78.233,45.5432))) * 43758.5453);
}
/**
* March the point p along the cast path c, and return a float2
* which is (density, depth); if the density is 0 no match was
* found in the given depth domain.
* @param point_ The origin point
* @param cast_ The cast vector
* @param max_ The maximum depth to step to
* @param step_ The increment to step in
* @return (denity, depth)
*/
float2 march_raycast(float3 point_, float3 cast_, float max_, float step_) {
float origin_ = point_;
float depth_ = 0;
float density_ = 0;
int steps = floor(max_ / step_);
for (int i = 0; (density_ <= 1) && (i < steps); ++i) {
float3 target_ = point_ + cast_ * i * step_ + rand(point_) * cast_ * step_;
density_ += march_geometry(target_);
depth_ = IF((depth_ == 0) && (density_ != 0), distance(point_, target_), depth_);
}
density_ = IF(density_ > 1, 1, density_);
return float2(density_, depth_);
}
/**
* Simple lighting; raycast from depth point to light source, and get density on path
* @param point_ The origin point on the render target
* @param cast_ The original cast (ie. camera view direction)
* @param raycast_ The result of the original raycast
* @param max_ The max distance to cast
* @param step_ The step increment
*/
float2 march_lighting(float3 point_, float3 cast_, float2 raycast_, float max_, float step_) {
float3 target_ = point_ + cast_ * raycast_[1];
float3 lcast_ = normalize(_sun - target_);
return march_raycast(target_, lcast_, max_, _lightStep);
}
struct fragmentInput {
float4 position : SV_POSITION;
float4 worldpos : TEXCOORD0;
float3 viewdir : TEXCOORD1;
};
struct fragmentOutput {
float4 color : SV_Target;
float zvalue : SV_Depth;
};
fragmentInput vert(appdata_base i) {
fragmentInput o;
o.position = mul(UNITY_MATRIX_MVP, i.vertex);
o.worldpos = mul(_Object2World, i.vertex);
o.viewdir = -normalize(WorldSpaceViewDir(i.vertex));
return o;
}
fragmentOutput frag(fragmentInput i) {
fragmentOutput o;
// Raycast
float2 output = march_raycast(i.worldpos, i.viewdir, _far, _step);
float2 light = march_lighting(i.worldpos, i.viewdir, output, _far, _step);
float lvalue = 1.0 - light[0];
float depth = output[1] / _far;
// Generate fragment color
float4 color = lerp(_light, _dark, lvalue);
// Debugging: Depth
float4 debug_depth = float4(depth, depth, depth, 1);
color = IF(_debugDepth, debug_depth, color);
// Debugging: Color
float4 debug_light = float4(lvalue, lvalue, lvalue, 1);
color = IF(_debugLight, debug_light, color);
// Always apply the depth map
color.a = output[0];
o.zvalue = IF(output[1] > 0, 0, 1);
o.color = IF(output[1] <= 0, 0, color);
return o;
}
ENDCG
}
}
}
(是的,我知道它非常复杂,但是很难将这种着色器简化为“简单的测试用例”来使用)
我很乐意接受任何答案,这是对上述着色器的修改,允许程序实体被遮挡/遮挡场景中的其他几何体,就像是“真实几何体”一样。
——
编辑:您可以通过使用与 raymarcher 相同的深度函数在场景中的其他几何体上显式设置深度值来获得此“工作”:
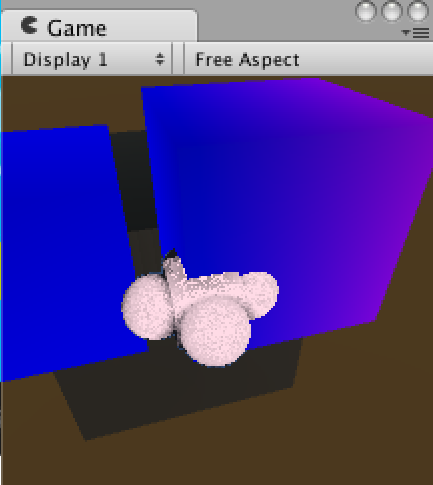
...但是,我仍然无法使用“标准”着色器使其与几何体一起正常工作。仍在寻找有效的解决方案......
最佳答案
看看你链接的项目,我看到的最重要的区别是他们的raycast march function使用传递引用参数返回名为 ray_pos
的片段位置.该位置似乎在对象空间中,因此它们 transform it using the view-projection matrix获取剪辑空间并读取深度值。
该项目还有一个compute_depth
功能,但 it looks pretty simple .
您的 march_raycast
函数已经在计算 target_
位置,所以你可以重构一下,应用 out
关键字将其返回给调用者,并在深度计算中使用它:
//get position using pass-by-ref
float3 ray_pos = i.worldpos;
float2 output = march_raycast(ray_pos, i.viewdir, _far, _step);
...
//convert position to clip space, read depth
float4 clip_pos = mul(UNITY_MATRIX_VP, float4(ray_pos, 1.0));
o.zvalue = clip_pos.z / clip_pos.w;
关于unity3d - 你如何在着色器中编写 z 深度?,我们在Stack Overflow上找到一个类似的问题: https://stackoverflow.com/questions/34532595/