我正在使用 ThreeJS 来“打开”和查看 3D 文件。
此刻我只能观察 3D 格式的 3D 图形。
如何用 3D 文件(obj 或 fbx)替换这个几何图形(立方体)?
在项目中,我放置了两个 3D 文件(一个 fbx 和另一个 obj),名称为 image。
我是否需要使用任何特定的加载程序来读取此类文件?
有人能帮我吗?
谢谢 !
DEMO
html
<canvas #canvas></canvas>
.ts @ViewChild('canvas') canvasRef: ElementRef;
renderer = new THREE.WebGLRenderer;
scene = null;
camera = null;
controls = null;
mesh = null;
light = null;
private calculateAspectRatio(): number {
const height = this.canvas.clientHeight;
if (height === 0) {
return 0;
}
return this.canvas.clientWidth / this.canvas.clientHeight;
}
private get canvas(): HTMLCanvasElement {
return this.canvasRef.nativeElement;
}
constructor() {
this.scene = new THREE.Scene();
this.camera = new THREE.PerspectiveCamera(35, 800/640, 0.1, 1000)
}
ngAfterViewInit() {
this.configScene();
this.configCamera();
this.configRenderer();
this.configControls();
this.createLight();
this.createMesh();
this.animate();
}
configScene() {
this.scene.background = new THREE.Color( 0xdddddd );
}
configCamera() {
this.camera.aspect = this.calculateAspectRatio();
this.camera.updateProjectionMatrix();
this.camera.position.set( -15, 10, 15 );
this.camera.lookAt( this.scene.position );
}
configRenderer() {
this.renderer = new THREE.WebGLRenderer({
canvas: this.canvas,
antialias: true,
alpha: true
});
this.renderer.setPixelRatio(devicePixelRatio);
this.renderer.setClearColor( 0x000000, 0 );
this.renderer.setSize(this.canvas.clientWidth, this.canvas.clientHeight);
}
configControls() {
this.controls = new OrbitControls(this.camera, this.canvas);
this.controls.autoRotate = false;
this.controls.enableZoom = true;
this.controls.enablePan = true;
this.controls.update();
}
createLight() {
this.light = new THREE.PointLight( 0xffffff );
this.light.position.set( -10, 10, 10 );
this.scene.add( this.light );
}
createMesh() {
const geometry = new THREE.BoxGeometry(5, 5, 5);
const material = new THREE.MeshLambertMaterial({ color: 0xff0000 });
this.mesh = new THREE.Mesh(geometry, material);
this.scene.add(this.mesh);
}
animate() {
window.requestAnimationFrame(() => this.animate());
// this.mesh.rotation.x += 0.01;
// this.mesh.rotation.y += 0.01;
this.controls.update();
this.renderer.render(this.scene, this.camera);
}
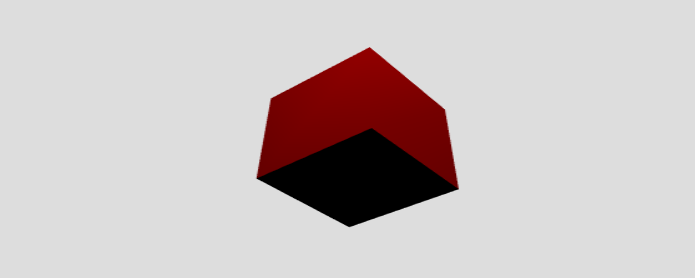
最佳答案
Do I need to use any specific loader to read this type of files?
是的。您必须使用
THREE.OBJLoader
和 THREE.FBXLoader
如果要加载 OBJ 或 FBX 文件。对于加载 OBJ 文件,工作流程如下所示:导入加载器:
import { OBJLoader } from 'three/examples/jsm/loaders/OBJLoader.js';
加载 Assets 并将其添加到您的场景中:const loader = new OBJLoader();
loader.load('https://threejs.org/examples/models/obj/tree.obj', object => {
this.scene.add(object);
});
顺便说一句:没有必要使用 npm 包 @avatsaev/three-orbitcontrols-ts
.您可以导入 OrbitControls
来自 three
包裹。import { OrbitControls } from 'three/examples/jsm/controls/OrbitControls.js';
关于three.js - 使用 ThreeJS 加载 3D 文件(fbx 和 obj),我们在Stack Overflow上找到一个类似的问题: https://stackoverflow.com/questions/64120991/