我正在将 USR-TCP232 LAN 模块用于嵌入式项目。
我有一个工具可以查询制造商制造的那些模块,效果很好。
但是,我希望它在我的 C# 代码中。所以,我决定自己做一个。我相信我已经很接近了,但我猜是一个小故障,这给我带来了困难,我需要有人来解释一下。
我可以发送一个 UDP 广播,我可以通过“WireShark”观察流量。
它与原始工具非常相似。但是,我无法在我的代码中接收网络上设备发送的应答数据。
C# 中的控制台应用程序
using System;
using System.Net.Sockets;
using System.Net;
using System.Text;
using System.Threading;
namespace UDPer
{
class Program
{
static void Main(string[] args)
{
UDPer udp = new UDPer();
udp.Starter();
ConsoleKeyInfo cki;
do
{
if (Console.KeyAvailable)
{
cki = Console.ReadKey(true);
switch (cki.KeyChar)
{
case 's':
udp.Send("0123456789012345678901234567890123456789");
break;
case 'x':
udp.Stop();
return;
}
}
Thread.Sleep(10);
} while (true);
}
}
class UDPer
{
private Socket udpSock;
private byte[] buffer;
public void Starter()
{
//Setup the socket and message buffer
udpSock = new Socket(AddressFamily.InterNetwork, SocketType.Dgram, ProtocolType.Udp);
udpSock.Bind(new IPEndPoint(IPAddress.Any, 0));
udpSock.EnableBroadcast = true;
buffer = new byte[1024];
udpSock.SetSocketOption(SocketOptionLevel.Socket, SocketOptionName.ReuseAddress, true);
//udpSock.ExclusiveAddressUse = false; // only if you want to send/receive on same machine.
//The socket must not be bound or connected.
//Start listening for a new message.
EndPoint newClientEP = new IPEndPoint(IPAddress.Any, 0);
udpSock.BeginReceiveFrom(buffer, 0, buffer.Length, SocketFlags.None, ref newClientEP, DoReceiveFrom, udpSock);
}
public void Stop()
{
try
{
udpSock.Close();
Console.WriteLine("Stopped listening");
}
catch { /* don't care */ }
}
private void DoReceiveFrom(IAsyncResult iar)
{
try
{
//Get the received message.
Socket recvSock = (Socket)iar.AsyncState;
recvSock.EnableBroadcast = true;
EndPoint clientEP = new IPEndPoint(IPAddress.Any, 0);
int msgLen = recvSock.EndReceiveFrom(iar, ref clientEP);
byte[] localMsg = new byte[msgLen];
Array.Copy(buffer, localMsg, msgLen);
//Start listening for a new message.
EndPoint newClientEP = new IPEndPoint(IPAddress.Any, 0);
udpSock.BeginReceiveFrom(buffer, 0, buffer.Length, SocketFlags.None, ref newClientEP, DoReceiveFrom, udpSock);
//Handle the received message
Console.WriteLine("Recieved {0} bytes from {1}:{2}",
msgLen,
((IPEndPoint)clientEP).Address,
((IPEndPoint)clientEP).Port);
//Do other, more interesting, things with the received message.
string message = Encoding.ASCII.GetString(localMsg);
Console.WriteLine("Message: {0} ", message);
}
catch (ObjectDisposedException)
{
//expected termination exception on a closed socket.
}
}
public void Send(string message)
{
UdpClient udp = new UdpClient(1500);
var ipEndPoint = new IPEndPoint(IPAddress.Broadcast, 1500);
byte[] data = Encoding.ASCII.GetBytes(message);
udp.Send(data, data.Length, ipEndPoint);
udp.Close();
}
}
}
Wireshark 捕获
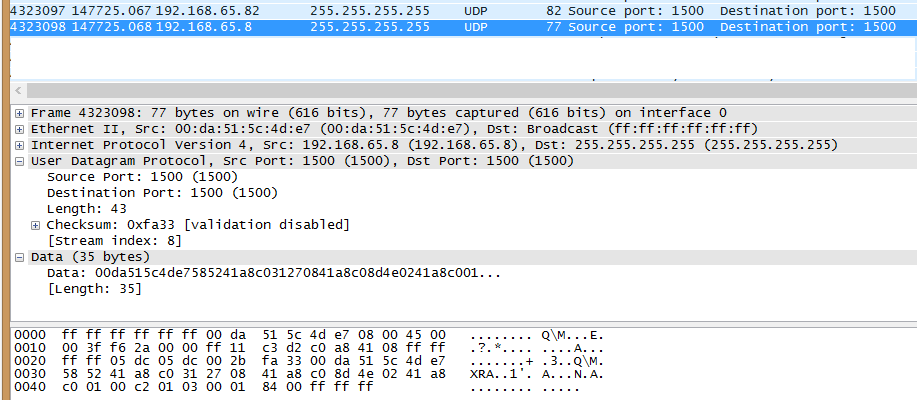
请注意: 此 Wireshark 捕获与原始工具完全相同。
一些定义:
我的 C# 应用程序所在的 PC:192.168.65.82
局域网模块IP地址:192.168.65.8
端口必须是 1500,这一切都可以。
发送有效载荷以查询 LAN 模块;
“0123456789012345678901234567890123456789”
所以,我一一尝试了很多不同的选择,但到目前为止还没有。
我在这里想念什么?
最佳答案
套接字总是有一个专用端口。通过 IPEndPoint
端口号为 0 并不意味着您接收到发送到任何端口的数据包。相反,您绑定(bind)到由底层服务提供商分配的端口。
udpSock.Bind(new IPEndPoint(IPAddress.Any, 0));
If you do not care which local port is used, you can create an IPEndPoint using 0 for the port number. In this case, the service provider will assign an available port number between 1024 and 5000
https://msdn.microsoft.com/en-us/library/system.net.sockets.socket.bind(v=vs.110).aspx
你需要使用
udpSock.Bind(new IPEndPoint(IPAddress.Any, 1500));
关于c# - 发送 UDP 广播以填充 USR-TCP232 LAN 模块信息,我们在Stack Overflow上找到一个类似的问题: https://stackoverflow.com/questions/39655932/