我有以下类(class)
public class Item
{
public int Id { get; set; }
public int ParentId { get; set; }
public string Content { get; set; }
public bool IsLastItem { get; set; }
}
假设我有以下模型,我想删除
IsLastItem = false
的项目并且没有 child 。在这种情况下 item4 和 item7 应该从列表中删除。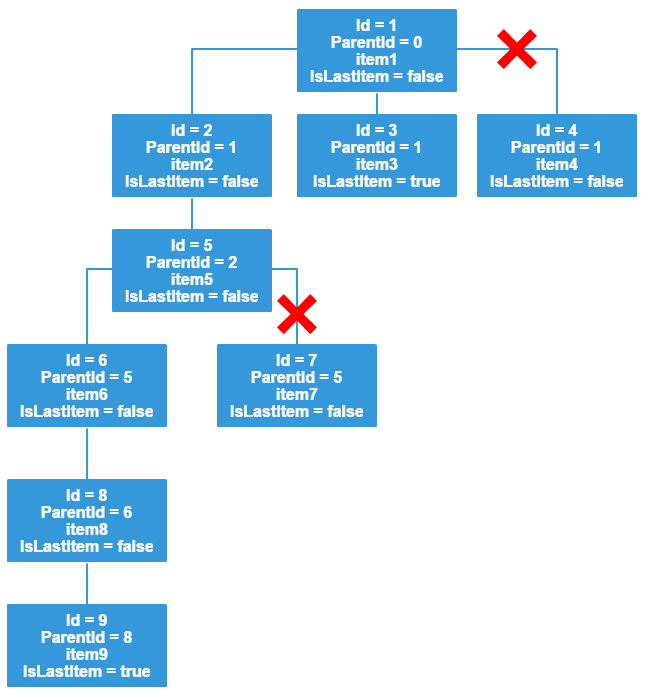
我从数据库中获取了我的模型列表,并像这样在代码块中对其进行了模拟
var items = new List<Item>
{
new Item
{
Id = 1,
ParentId = 0,
Content = "item1",
IsLastItem = false
},
new Item
{
Id = 2,
ParentId = 1,
Content = "item2",
IsLastItem = false
},
new Item
{
Id = 3,
ParentId = 1,
Content = "item3",
IsLastItem = true
},
new Item
{
Id = 4,
ParentId = 1,
Content = "item4",
IsLastItem = false
},
new Item
{
Id = 5,
ParentId = 2,
Content = "item5",
IsLastItem = false
},
new Item
{
Id = 6,
ParentId = 5,
Content = "item6",
IsLastItem = false
},
new Item
{
Id = 7,
ParentId = 5,
Content = "item7",
IsLastItem = false
},
new Item
{
Id = 8,
ParentId = 6,
Content = "item8",
IsLastItem = true
},
new Item
{
Id = 9,
ParentId = 8,
Content = "item9",
IsLastItem = true
}
};
最佳答案
像这样的平面列表对于这些类型的操作来说并不是最佳的——如果你能以某种树结构取回列表可能会很好(如果你在 2016 年,可以使用 FOR XML
或 JSON 从 SQL 中返回它)首先,您可以更轻松地遍历树。
另请注意,您的示例数据未设置 IsLastItem ...
照原样,您必须至少迭代两次,如下所示:
items.RemoveAll(x => x.IsLastItem == false &&
items.Any(y => y.ParentId == x.Id) == false);
您是说要删除
IsLastItem
中的所有项目是 false 并且没有至少一个项目的父 id 是该项目的 id。
关于C#如何从列表中删除特定节点,我们在Stack Overflow上找到一个类似的问题: https://stackoverflow.com/questions/42145819/